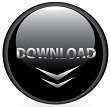
combine the above elements into a figure - fig.create a layout object layout to set the title before animation (optional).This is also done with a Scatter object in Plotly create a graph_object for our scatter plot - points.Because our animation will be made up of more than one plot (a scatter plot and a line plot) we will use aph_objects for the elements of our figure. Now we will walk through creating the same plot with plotly. # Set up formatting for the movie files from matplotlib.animation import writers Writer = writers writer = Writer(fps=12.5) # save an mp4 of the animation animation.save('matplotlib.mp4', writer=writer) use the writer to write an mp4 file to disc.instantiate a writer to define the output file parameters.Because matplot lib is generating rasterized images for each frame we will save this plot to mp4 file. Saving the animation with matplotlib is arguably the most confusing part. interval: the delay in milliseconds between frames.This is equal to the total number of frames in the final animation. frames: the total number of times to call the above function.func: the function to call to update each frame.fig: the figure object on which to apply the animation.The attributes of FuncAnimation attributes used here are: # run the linear regression and store the m and b values ms, bs = linear_regression(X.ravel(), y, epochs=200, learning_rate=0.01) # build the lines, mx + b for all ms X's and b's y_lines = (ms * X + bs).T # transpose for easier indexing To generate the data for the animation of the line fitting, we feed our X data into the linear_regression function and calculate the sequential lines for plotting. def linear_regression(X, y, m_current=0, b_current=0, epochs=1000, learning_rate=0.0001): N = float(len(y)) ms = # a place to store our m values bs = # a place to store our b values for i in range(epochs): y_current = (m_current * X) + b_current cost = sum() / N m_gradient = -(2/N) * sum(X * (y - y_current)) b_gradient = -(2/N) * sum(y - y_current) m_current = m_current - (learning_rate * m_gradient) b_current = b_current - (learning_rate * b_gradient) ms.append(m_current) bs.append(b_current) return ms, bs Predict This isn’t necessary to understand for the animation, but here is the regression function. I instead borrowed the code from Joseph Bautista’s post Linear Regression Using Gradient Descent in 10 Lines of Code and modified it to return a list of of the slopes and intercepts for each of iteration of the fit. Then, you should be able to update the example.txt file with new coordinates.We can’t use statsmodels or sklearn’s LinearRegression models because we need to store each iteration of the regression, not just the final fit line. The result of running this graph should give you a graph as usual. We run the animation, putting the animation to the figure (fig), running the animation function of "animate," and then finally we have an interval of 1000, which is 1000 milliseconds, or one second.

Then: ani = animation.FuncAnimation(fig, animate, interval=1000) We open the above file, and then store each line, split by comma, into xs and ys, which we'll plot. We read data from an example file, which has the contents of: 1,5 What we're doing here is building the data and then plotting it. Graph_data = open('example.txt','r').read() Now we write the animation function: def animate(i):

Next, we'll add some code that you should be familiar with if you're following this series: e('fivethirtyeight')
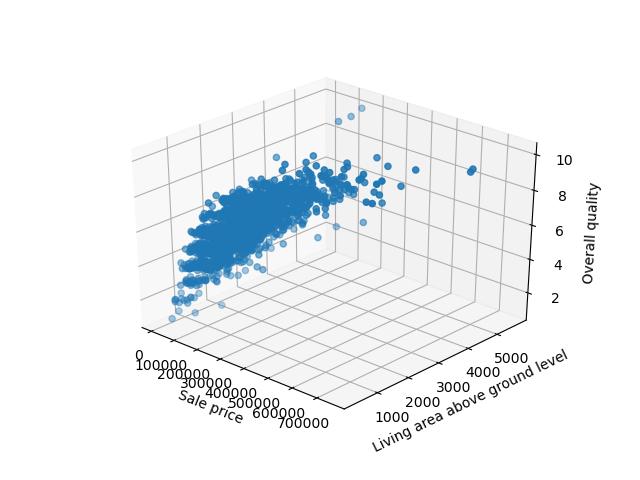
This is the module that will allow us to animate the figure after it has been shown. Here, the only new import is the matplotlib.animation as animation. To start: import matplotlib.pyplot as plt To do this, we use the animation functionality with Matplotlib. You may want to use this for something like graphing live stock pricing data, or maybe you have a sensor connected to your computer, and you want to display the live sensor data. In this Matplotlib tutorial, we're going to cover how to create live updating graphs that can update their plots live as the data-source updates.
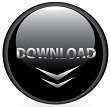